General Overview
Welcome to my first tutorial on creating an entity component system!
In this first tutorial we will be covering the basic setup of entity handles.
We will start here because they are the core of the entire system we will build and don’t need much else to support them.
We will work on providing a way to get the entity handles directly from the entity in an efficient way so we do not generate lots of garbage that needs to be collected in our Entity Component System (ECS) using C# using Test Driven Development (TDD).
This tutorial covers programming the creation of entity handles for Uber Entity Component System using Test Driven Development.
Goals
Project Setup
Setup Our DLL project Jump to Project Setup
Testing Setup
Setup the project to run and contain all the tests for our library from. Jump to Testing Setup
Create Tests
Create the tests that define the scope of this unit of work. Jump to Tests
Program!
Impliment the code that fulfills the defined scope. Jump To Expansions
Project Setup
Let's get started the first thing we need to do is setup our main code project!
Starting out we need to create a new Class Library project to do this select File > New > Project
We are going to be making a Class Library (.NET Framework) we will be choosing this instead of .NET Standard because we want it to be more compatible with everything. I develop in a folder on my C drive called dev so I will put it in C:\dev\Unity3D\Projects how ever you may like it somewhere else so pick what makes sense for your development environment. I will call my library UberEnityComponentSystem below is a screen shot of what your window should look like if your doing exactly the same.
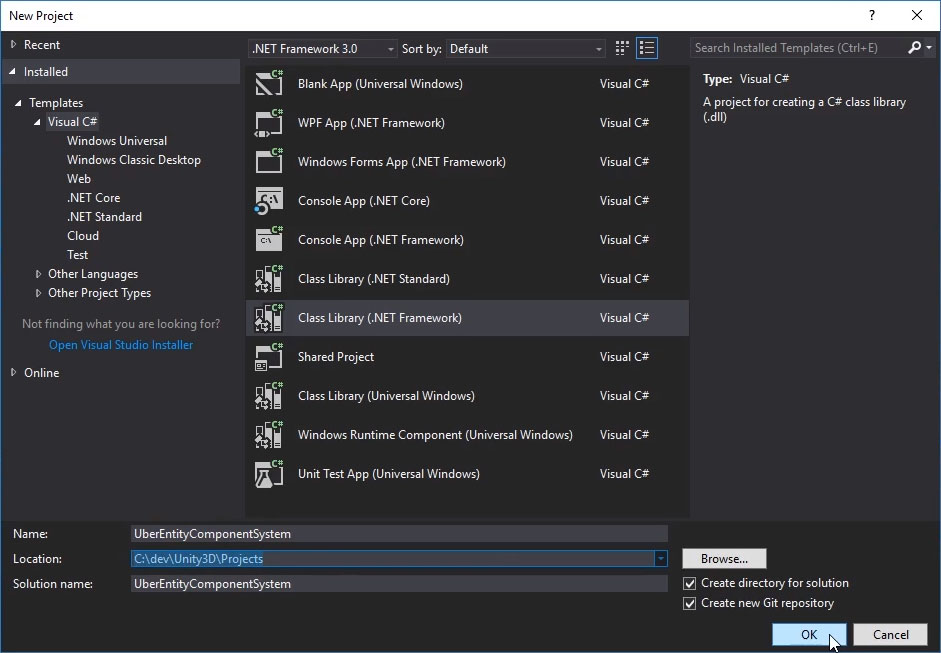
The next thing we need to do is change the Target Framework to something that is compatible with unity because we want to be able to use this library in unity. If your not going to be targeting unity feel free to select what ever framework you feel best suits your needs.
To change the Target Framework right click our new Class Library project in the solution explorer and then select UberEntityComponentSystem > Properties
As we created a Class Library (.NET Framework) some version of .NET Framework will be selected in the Target Framework dropdown. Lets change that to Unity 3.5 .net full Base Class Libraries. If you do not have this then your unity install or visual studio install did not work.
Note: You only need to do this step if you want to use the final lib in Unity3D
Testing Setup
Finally lets make an Entity.cs file so we can setup our testing.
// File: Entity.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace UberEntityComponentSystem
{
public class Entity
{
public void Test()
{
}
}
}
Visual Studio allows us to create a test project in a very simple way by just Right Clicking our Method then selecting Create Unit Tests
To properly generate our unit test project select the following settings:
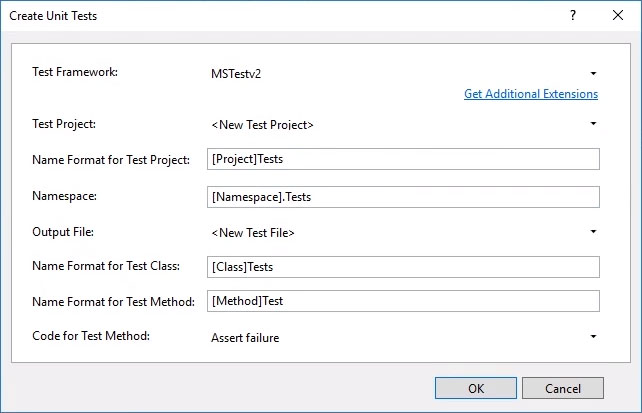
This should create a project called UberEntityComponentSystemTests with a file called EntityTests.cs in it.
It starts with a basic test that asserts a fail state without actually doing any testing.
Lets make a simple instantiation test by changing the starter test file to something like this:
// File: EntityTests.cs
using Microsoft.VisualStudio.TestTools.UnitTesting;
using UberEntityComponentSystem;
using System;
using System.Collections.Generic;
using System.Text;
namespace UberEntityComponentSystem.Tests
{
[TestClass()]
public class EntityTests
{
[TestMethod()]
public void InstantiationTest()
{
new Entity();
}
}
}
In Visual Studio we can run our tests by clicking Test > Run > All Tests or pressing Ctrl+R and then A, Pressing L after Ctrl+R will run what ever the last test we ran was also.
After running this simple test we should see it in passed tests in the Test Explorer window.
Create Tests
Now that we have our testing project up we can move on to actually making our first tests.
In this tutorial I want to build out the following tests:
- Entity Phase
When an entity is recycled we will call it out of phase and break all links to it that we had. So let's write a test for this.
We can start with the method to hold the test:
[TestMethod()]
public void PhaseTest()
{
//Testing code here
}
We add this into our EntityTests class with the Decorator [TestMethod()] so that it gets detected as something we want to run as a test. Right now this test does not do anything so let's change that. First we need to create our entities to test with.